Selenium Grid is a tool that distributes the tests across multiple physical or virtual machines so that we can execute scripts in parallel (simultaneously). It dramatically accelerates the testing process across browsers and across platforms by giving us quick and accurate feedback.
Selenium Grid allows us to execute multiple instances of WebDriver or Selenium Remote Control tests in parallel which uses the same code base, hence the code need NOT be present on the system they execute. The selenium-server-standalone package includes Hub, WebDriver, and Selenium RC to execute the scripts in grid.
Selenium Grid has a Hub and a Node.
- Hub − The hub can also be understood as a server which acts as the central point where the tests would be triggered. A Selenium Grid has only one Hub and it is launched on a single machine once.
- Node − Nodes are the Selenium instances that are attached to the Hub which execute the tests. There can be one or more nodes in a grid which can be of any OS and can contain any of the Selenium supported browsers.
Architecture
The following diagram shows the architecture of Selenium Grid.

Working with Grid
In order to work with the Grid, we need to follow certain protocols. Listen below are the major steps involved in this process −
- Configuring the Hub
- Configuring the Nodes
- Develop the Script and Prepare the XML File
- Test Execution
- Result Analysis
Let us discuss each of these steps in detail.
Configuring the Hub
Step 1 − Download the latest Selenium Server standalone JAR file from http://docs.seleniumhq.org/download/. Download it by clicking on the version as shown below.
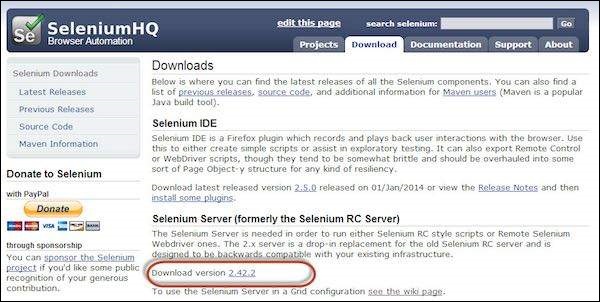
Step 2 − Start the Hub by launching the Selenium Server using the following command. Now we will use the port '4444' to start the hub.
Note − Ensure that there are no other applications that are running on port# 4444.
java -jar selenium-server-standalone-2.25.0.jar -port 4444 -role hub -nodeTimeout 1000

Step 3 − Now open the browser and navigate to the URL http//localhost:4444 from the Hub (The system where you have executed Step#2).
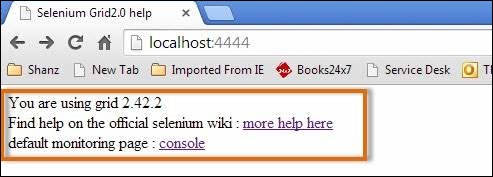
Step 4 − Now click on the 'console' link and click 'view config'. The config of the hub would be displayed as follows. As of now, we haven't got any nodes, hence we will not be able to see the details.
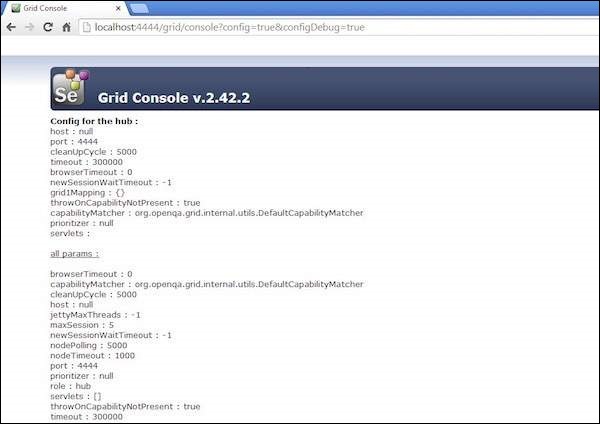
Configuring the Nodes
Step 1 − Logon to the node (where you would like to execute the scripts) and place the 'selenium-server-standalone-2.42.2' in a folder. We need to point to the selenium-server-standalone JAR while launching the nodes.
Step 2 − Launch FireFox Node using the following below command.
java -jar D:\JAR\selenium-server-standalone-2.42.2.jar -role node -hub http://10.30.217.157:4444/grid/register -browser browserName = firefox -port 5555
Where,
D:\JAR\selenium-server-standalone-2.42.2.jar = Location of the Selenium Server Standalone Jar File(on the Node Machine)
http://10.30.217.157:4444 = IP Address of the Hub and 4444 is the port of the Hub
browserName = firefox (Parameter to specify the Browser name on Nodes)
5555 = Port on which Firefox Node would be up and running.

Step 3 − After executing the command, come back to the Hub. Navigate to the URL - http://10.30.217.157:4444 and the Hub would now display the node attached to it.

Step 4 − Now let us launch the Internet Explorer Node. For launching the IE Node, we need to have the Internet Explorer driver downloaded on the node machine.
Step 5 − To download the Internet Explorer driver, navigate to http://docs.seleniumhq.org/download/ and download the appropriate file based on the architecture of your OS. After you have downloaded, unzip the exe file and place in it a folder which has to be referred while launching IE nodes.

Step 6 − Launch IE using the following command.
C:\>java -Dwebdriver.ie.driver = D:\IEDriverServer.exe -jar D:\JAR\selenium-server-standalone-2.42.2.jar -role webdriver -hub http://10.30.217.157:4444/grid/register -browser browserName = ie,platform = WINDOWS -port 5558
Where,
D:\IEDriverServer.exe = The location of the downloaded the IE Driver(on the Node Machine)
D:\JAR\selenium-server-standalone-2.42.2.jar = Location of the Selenium Server Standalone Jar File(on the Node Machine)
http://10.30.217.157:4444 = IP Address of the Hub and 4444 is the port of the Hub
browserName = ie (Parameter to specify the Browser name on Nodes)
5558 = Port on which IE Node would be up and running.

Step 7 − After executing the command, come back to the Hub. Navigate to the URL - http://10.30.217.157:4444 and the Hub would now display the IE node attached to it.

Step 8 − Let us now launch Chrome Node. For launching the Chrome Node, we need to have the Chrome driver downloaded on the node machine.
Step 9 − To download the Chrome Driver, navigate to http://docs.seleniumhq.org/download/ and then navigate to Third Party Browser Drivers area and click on the version number '2.10' as shown below.
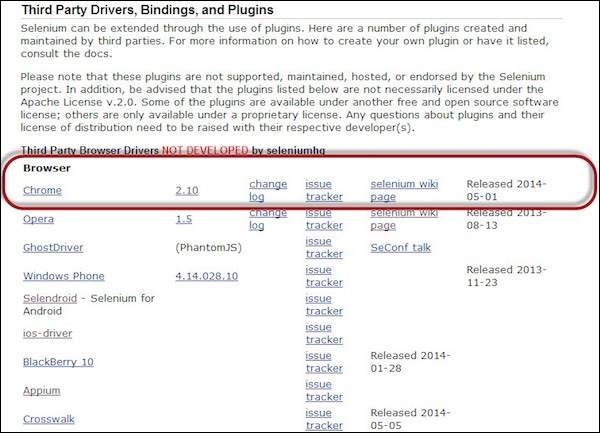
Step 10 − Download the driver based on the type of your OS. We will execute it on Windows environment, hence we will download the Windows Chrome Driver. After you have downloaded, unzip the exe file and place it in a folder which has to be referred while launching chrome nodes.

Step 11 − Launch Chrome using the following command.
C:\>java -Dwebdriver.chrome.driver = D:\chromedriver.exe -jar D:\JAR\selenium-server-standalone-2.42.2.jar -role webdriver -hub http://10.30.217.157:4444/grid/register -browser browserName = chrome, platform = WINDOWS -port 5557
Where,
D:\chromedriver.exe = The location of the downloaded the chrome Driver(on the Node Machine)
D:\JAR\selenium-server-standalone-2.42.2.jar = Location of the Selenium Server Standalone Jar File(on the Node Machine)
http://10.30.217.157:4444 = IP Address of the Hub and 4444 is the port of the Hub
browserName = chrome (Parameter to specify the Browser name on Nodes)
5557 = Port on which chrome Node would be up and running.
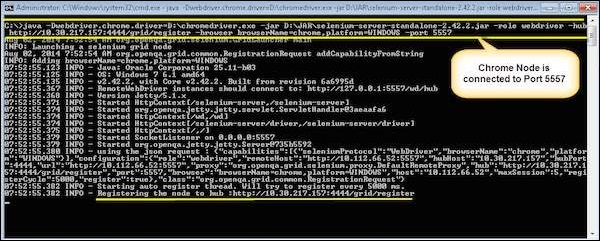
Step 12 − After executing the command, come back to the Hub. Navigate to the URL - http://10.30.217.157:4444 and the Hub would now display the chrome node attached to it.
